Clusters Simulation
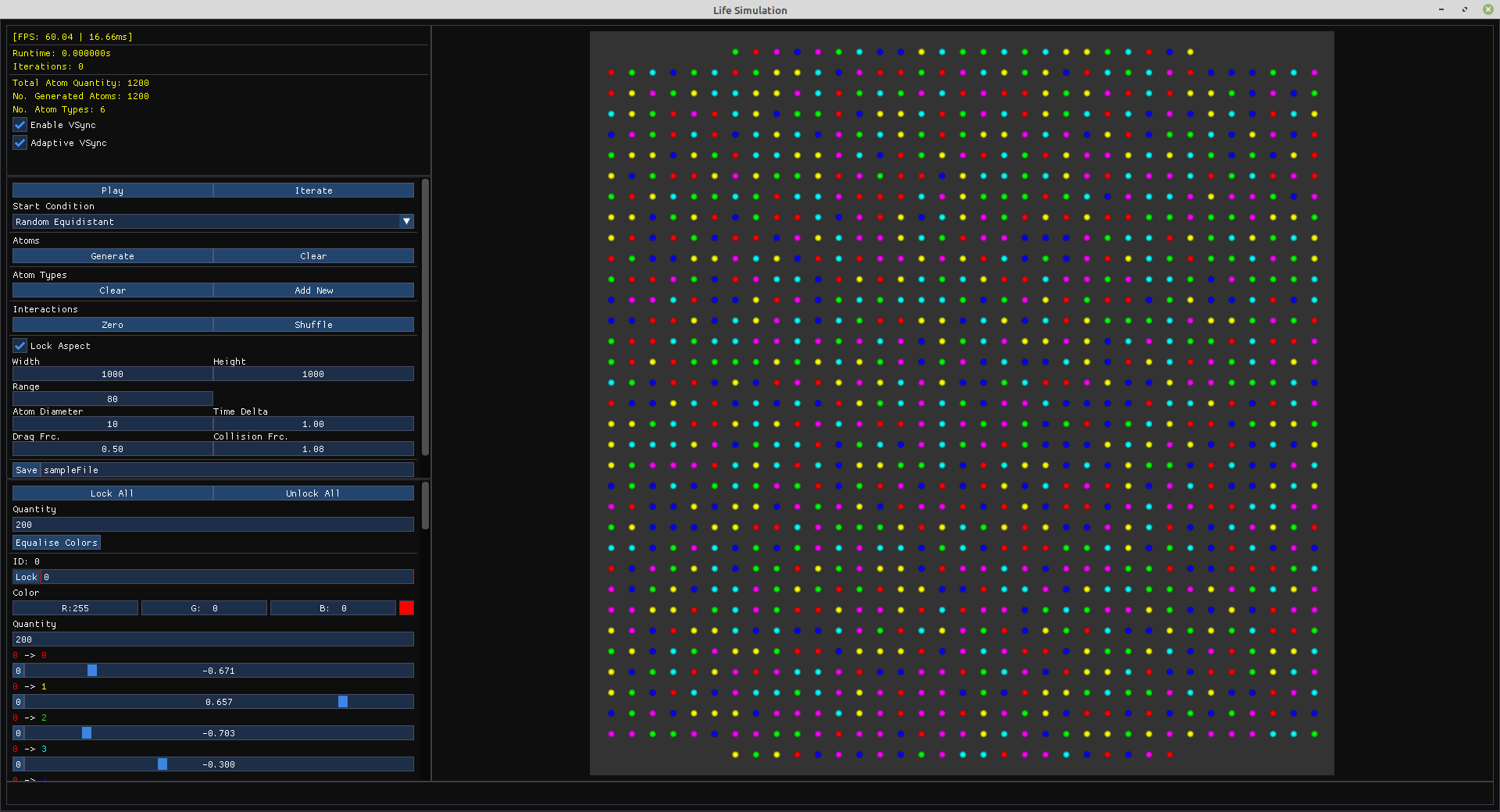
The Clusters Simulation application is a C++ project based on Jeffrey Ventrella's Clusters Simulation, and inspired by this YouTube video.
I primarily made this application to learn C++, so while some of the code itself is messy and poorly optimized, I am still quite proud of the result. The application uses compute shaders to run the simulation more efficiently (cmake builds two executables, one for CPU and one for GPU, so they can be compared). Using compute shaders gives me an increase in performance of up to 10 times, compared to using CPU only.
This simulation involves creating several Atoms and Atom Types, and defining how Atoms of different Types interact (either attracting, repelling, or completely ignoring each other). Different configurations create emergent behaviour which resemble life-like structures (in particular, really simple cells).
See below for a simple JavaScript version of this simulation.
Libraries
Dear ImGui | GUI library for creating UI widgets on top of a window. Generally used for debug windows, but can be used for application layout (as I often do). |
glad | Library for interfacing with the GPU through OpenGL. |
glm | Utility library with useful data structures and functions for graphics and 3D maths, matching those seen in glsl (e.g. vec3, mat4, normalize). |
SDL2 | GUI library for C++, used to create the main application window and handle user input events. Often used for games and simulations. |
Examples
Below are examples of the same configuration being run using each of the different starting conditions:
Random+Equidistant | Random |
---|---|
Equidistant | Rings |
Structures
Here I provide some examples of the emergent behaviour that can be seen in this simulation.
Cells
I denote cells as emergent structures which maintain stable ring-like forms. Many cells will be robust, even in the presence of distruptive chasers, though some may be fragile and break apart after only a short time. Occasionally they oscillate between multiple states, and there is some overlap with spinners.
Terms:
- Tier-N - Tier of the cell, where N is number of Atom Types a cell is composed of.
- Oscillator - Oscillates between two or more patterns over time
Tier-2 Cells | |||
---|---|---|---|
![]() |
|||
![]() |
|||
Tier-3 Cells | |||
![]() |
|||
![]() |
|||
Tier-2 Oscillators | |||
![]() |
![]() |
||
Tier-3 Oscillators | |||
![]() |
![]() |
![]() |
![]() |
Chasers
I denote chasers as emergent structures which persistantly move. Usually they consist of a head and a tail. Most of the time chasers will move erratically, often colliding into each other and either combining into a larger chaser, breaking apart, or even combining into a stable cell.
Terms:
- Tier-H-T - Tier of the chaser, where H is the number of heads, and T is the number of tails.
- Head - Usually orb-like structures at the front of the chaser.
- Tail - Longer trails of Atoms which chase after the head.
Tier-4 Chasers |
---|
![]() |
Spinners
I denote spinners as emergent structures which appear to contain nodes which spin around each other in an orbiting-like manner. These are more common when there are a lot of atom types.
Terms:
- Tier-N - Tier of the spinner, where N is the number of nodes.
- Node - Usually orb-shaped cluster of a single Atom Type composing one part of the spinner.
Tier-3 Spinners | |||
---|---|---|---|
![]() |
![]() |
![]() |
![]() |
Web App
Proximity Repulsion Force (Collision):